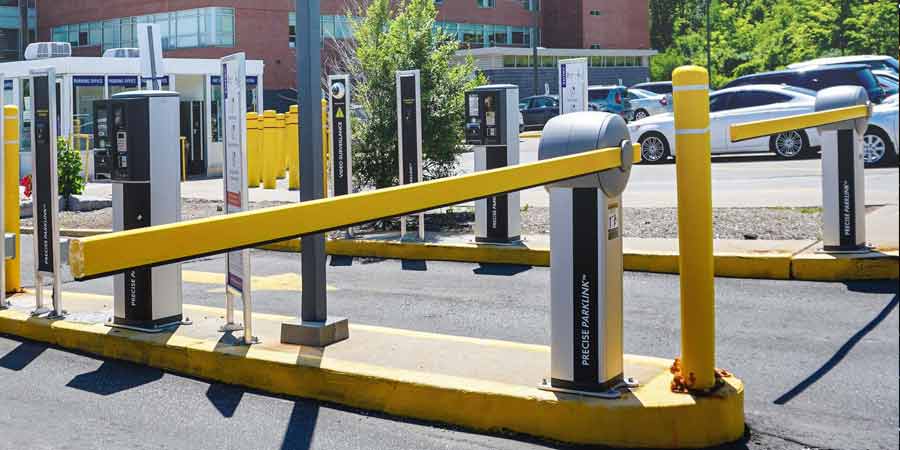
To ensure the class property will never be modified/changed by itself or children or external calls we can now use a readonly
prefix to class property declaration like so: public readonly string $propertyName = 'thisValueWillNeverChange;
. The only place when we could override the readonly variable is inside the class constructor.