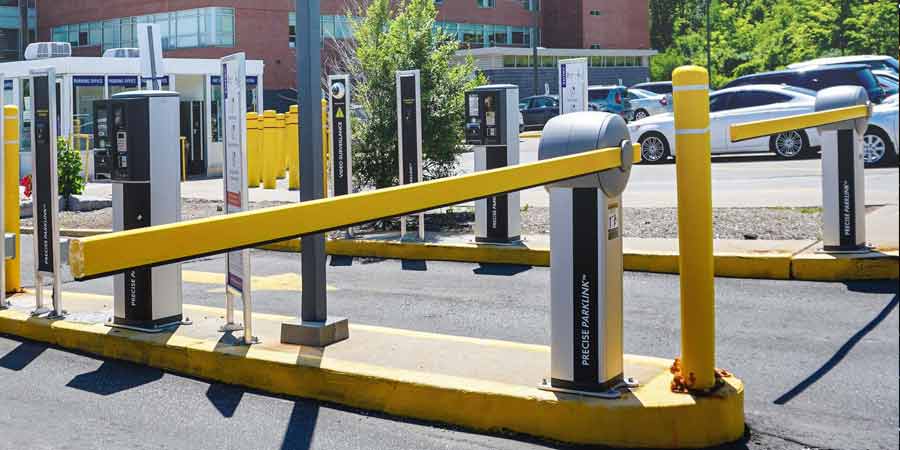
To ensure the class property will never be modified/changed by itself or children or external calls we can now use a readonly
prefix to class property declaration like so: public readonly string $propertyName = 'thisValueWillNeverChange;
. The only place when we could override the readonly variable is inside the class constructor.
A readonly
property can only be initialized once, and only from the scope where it has been declared. Any other assignment or modification of the property will result in an Error
exception.
lass Test {
public readonly string $prop;
public function __construct(string $prop) {
// Legal initialization.
$this->prop = $prop;
}
}
$test = new Test("foobar");
// Legal read.
var_dump($test->prop); // string(6) "foobar"
// Illegal reassignment. It does not matter that the assigned value is the same.
$test->prop = "foobar";
// Error: Cannot modify readonly property Test::$prop
This variant is not allowed, as the initializing assignment occurs from outside the class:
class Test {
public readonly string $prop;
}
$test = new Test;
// Illegal initialization outside of private scope.
$test->prop = "foobar";
// Error: Cannot initialize readonly property Test::$prop from global scope
More about PHP 8.1
Read about all PHP 8.1 features and changes in here.