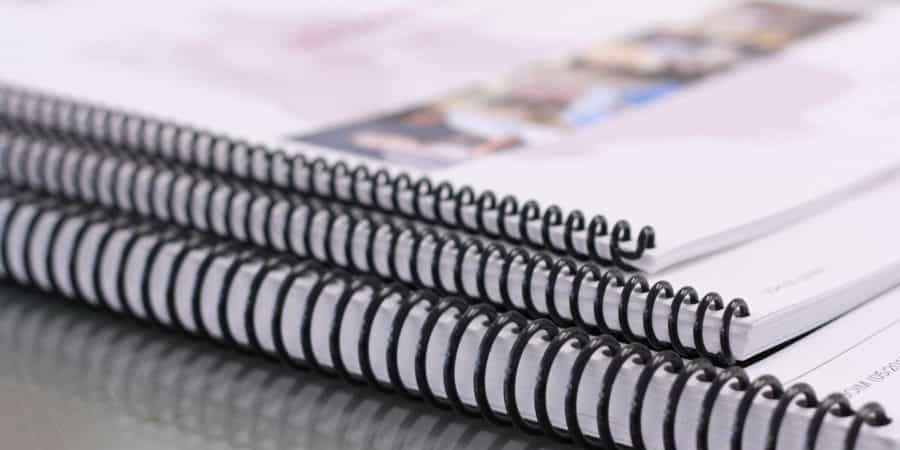
MySQLi is gettng a feature to bind arrays to execute($array)
method in single call. This would apply to standard int
index based arrays and associative arrays.
Added a new optional argument to mysqli_stmt::execute()
same as PDO
does with PDOStatement::execute()
. The goal of this proposal is to simplify mysqli usage with a simple fix that does not require major refactoring.
This proposal tries to address the following mysqli limitations:
/ mysqli can only bind by reference and each variable needs to be passed as a separate argument.
$id = 1;
$name = trim(' Dharman ');
$stmt = $mysqli->prepare('INSERT INTO users(id, name) VALUES(?,?)');
$stmt->bind_param('ss', $id, $name);
$stmt->execute();
// The following would fail and throw an error
$stmt = $mysqli->prepare('INSERT INTO users(id, name) VALUES(?,?)');
$stmt->bind_param('ss', 1, trim(' Dharman '));
$stmt->execute();
// Binding an array can be very confusing
$arr = [2,3,5,8,13];
$stmt = $mysqli->prepare('SELECT name FROM users WHERE id IN ('.str_repeat('?,', count($arr) - 1) . '?)');
$stmt->bind_param(str_repeat('s', count($arr)), ...$arr);
$stmt->execute();
// SOLUTION: bind in execute
// it is now possible to bind by value
$stmt = $mysqli->prepare('INSERT INTO users(id, name) VALUES(?,?)');
$stmt->execute([1, trim(' Dharman ')]);
// binding an array becomes less of a chore
$arr = [2,3,5,8,13];
$stmt = $mysqli->prepare('SELECT name FROM users WHERE id IN ('.str_repeat('?,', count($arr) - 1) . '?)');
$stmt->execute($arr);
More about PHP 8.1
Read about all PHP 8.1 features and changes in here.